@Blog{zip358.com}
日常日誌からプログラムやYOUTUBER紹介、旅日記まで日々更新中です。
DropFTPを配布。
2018.12.01
ドップして一つのファイルを転送するソフトを作りました。
こんなのどうしているのかと疑問を持つ人もいると思いますが
業務上、こんなソフトが要るという会社などもいるのではないかと
思いで作りました。
ダウンロードはこちらから
https://zip358.com/tool/DropFTP.zip
ソースコードは下記になります。
※FTP部分はWinSCPのライブラリを使用しています。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using WinSCP;
namespace dropFTP
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void upbtn_Click(object sender, EventArgs e)
{
String err = "";
if (hostText.Text == "") {
err += "ホスト名が設定されていませんn";
}
if (idText.Text == "")
{
err += "IDが設定されていませんn";
}
if (passText.Text == "")
{
err += "passが設定されていませんn";
}
if (remText.Text == "")
{
err += "アップロード場所が設定されていませんn";
}
if (uplab.Text == "")
{
err += "アップロードファイルが設定されていませんn";
}
var RadioGroup = groupFTP.Controls.OfType<RadioButton>().SingleOrDefault(rb => rb.Checked == true);
if (RadioGroup == null) {
err += "アップロード環境が設定されていませんn";
}
if (err != "")
{
MessageBox.Show(err);
}
else {
if (RadioGroup.Text == "FTP") {
upFTP();
}
if (RadioGroup.Text == "SFTP")
{
upSFTP();
}
}
}
private int upFTP() {
try
{
// Setup session options
SessionOptions sessionOptions = new SessionOptions
{
Protocol = Protocol.Ftp,
HostName = hostText.Text,
UserName = idText.Text,
Password = passText.Text,
PortNumber =int.Parse(portText.Text)
};
using (Session session = new Session())
{
// Connect
session.Open(sessionOptions);
// Upload files
TransferOptions transferOptions = new TransferOptions();
transferOptions.TransferMode = TransferMode.Binary;
TransferOperationResult transferResult;
if (remText.Text.EndsWith("/"))
{
transferResult = session.PutFiles(@uplab.Text, remText.Text, false, transferOptions);
}
else
{
transferResult = session.PutFiles(@uplab.Text, remText.Text + "/", false, transferOptions);
}
// Throw on any error
transferResult.Check();
// Print results
foreach (TransferEventArgs transfer in transferResult.Transfers)
{
MessageBox.Show("アップロードしました");
}
}
return 0;
}
catch (Exception e)
{
MessageBox.Show("Error: {0}" + e);
return 1;
}
}
private int upSFTP()
{
try
{
// Setup session options
SessionOptions sessionOptions = new SessionOptions
{
Protocol = Protocol.Sftp,
HostName = hostText.Text,
UserName = idText.Text,
Password = passText.Text,
PortNumber = int.Parse(portText.Text),
GiveUpSecurityAndAcceptAnySshHostKey = true
};
using (Session session = new Session())
{
// Connect
session.Open(sessionOptions);
// Upload files
TransferOptions transferOptions = new TransferOptions();
transferOptions.TransferMode = TransferMode.Binary;
TransferOperationResult transferResult;
if (remText.Text.EndsWith("/")) {
transferResult = session.PutFiles(@uplab.Text, remText.Text, false, transferOptions);
} else {
transferResult = session.PutFiles(@uplab.Text, remText.Text + "/", false, transferOptions);
}
// Throw on any error
transferResult.Check();
// Print results
foreach (TransferEventArgs transfer in transferResult.Transfers)
{
MessageBox.Show("アップロードしました");
}
}
return 0;
}
catch (Exception e)
{
MessageBox.Show("Error: {0}" + e);
return 1;
}
}
private void Form1_DragDrop(object sender, DragEventArgs e) {
//e.Effect = DragDropEffects.Copy;
string[] fileName = (string[])e.Data.GetData(DataFormats.FileDrop, false);
uplab.Text = fileName[0];
}
private void Form1_DragEnter(object sender, DragEventArgs e)
{
e.Effect = DragDropEffects.Copy;
}
private void radioSFTP_CheckedChanged(object sender, EventArgs e)
{
portText.Text = "22";
}
private void radioFTP_CheckedChanged(object sender, EventArgs e)
{
portText.Text = "21";
}
private void Form1_Load(object sender, EventArgs e)
{
this.FormBorderStyle = FormBorderStyle.FixedSingle;
this.MaximumSize = this.Size;
this.MinimumSize = this.Size;
}
}
}
著者名
@taoka_toshiaki
※この記事は著者が30代前半に書いたものです.
Profile
高知県在住の@taoka_toshiakiです、記事を読んで頂きありがとうございます.
数十年前から息を吸うように日々記事を書いてます.たまに休んだりする日もありますがほぼ毎日投稿を心掛けています😅.
SNSも使っています、フォロー、いいね、シェア宜しくお願い致します🙇.
SNS::@taoka_toshiaki
タグ
358, Collections, com, ComponentModel, data, Drawing, DropFTP, Forms, FTP, Generic, Linq, System, Tasks, Text, Threading, tool, using, Wi, Windows, WinSCP, zip, コード, こちら, ソース, ソフト, ダウンロード, ドップ, ファイル, ライブラリ, 一つ, 下記, 人, 会社, 使用, 業務, 疑問, 転送, 部分, 配布,
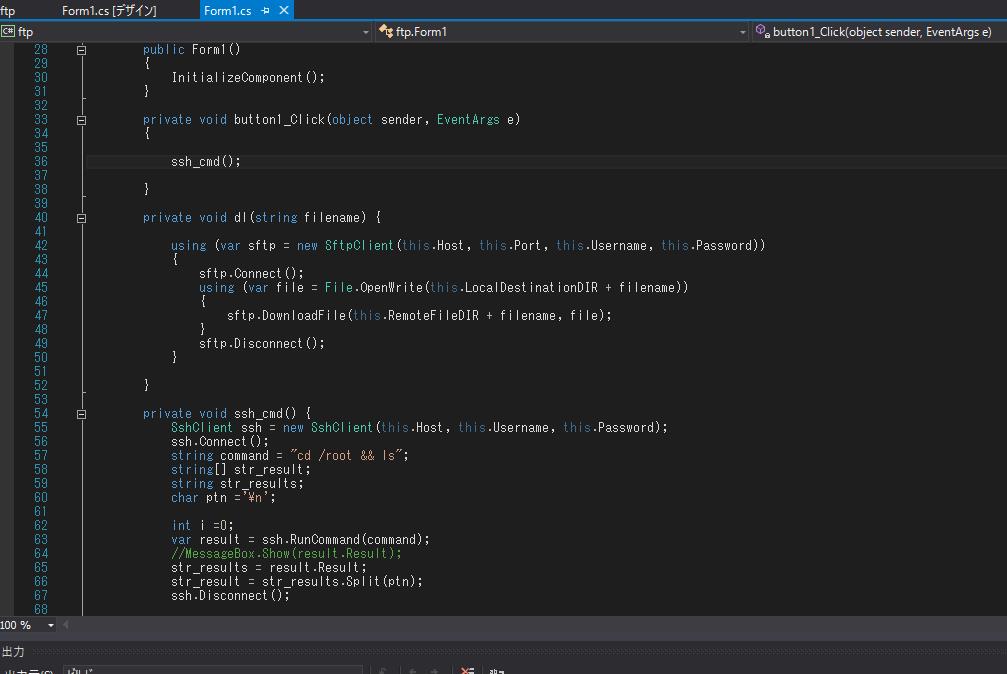
SSH.NETでSFTPとSSHの接続を確立させるまでの過程。
2016.12.11
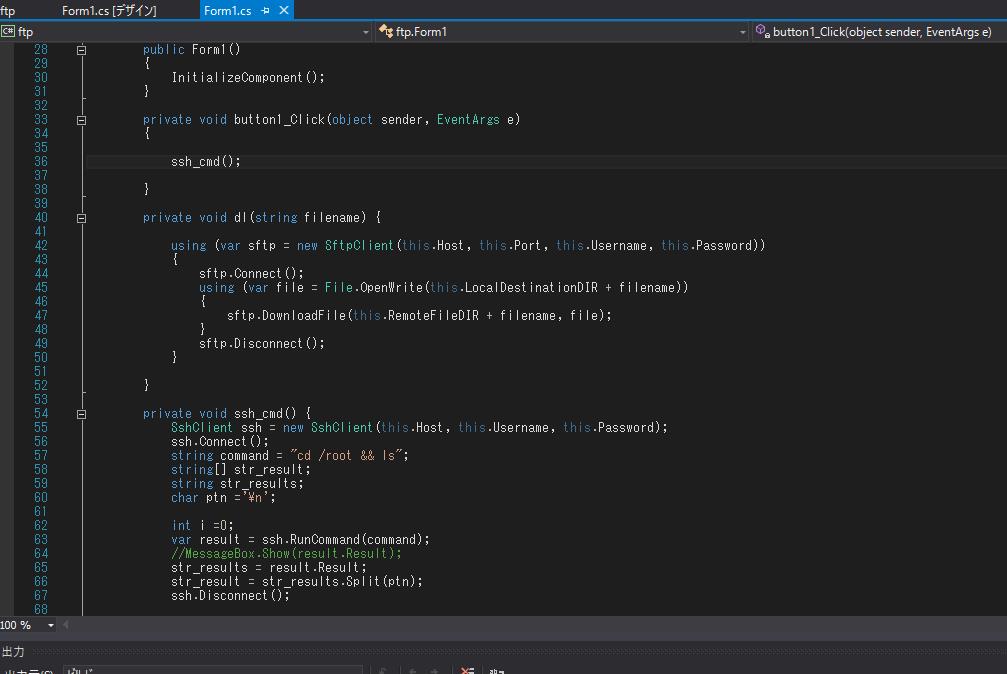
SSH.NETでSFTPとSSHの接続を確立させるまでの過程。
ソースコードは下記です、あくまでも触りなのでココからご自分で考えて作り変えてください。ちなみにSSH.NETのライブラリを入手するには拡張機能からNuGet Package Managerという拡張機能を追加するとツールのNuGetパッケージマネージャーが現れるので、ソリューションのNuGetパッケージ管理からSSH.NETと検索しインストールすることによりライブラリが使用できます。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.IO;
using Renci.SshNet;
using Renci.SshNet.Common;
using Renci.SshNet.Sftp;
namespace ftp
{
public partial class Form1 : Form
{
String Host = "168.192.11.1";
int Port = 22;
String RemoteFileDIR = "/root/";
String LocalDestinationDIR = "C:\\Users\\hoge\\Documents\\BACKUP\\";
String Username = "admin";
String Password = "hogehoge";
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
ssh_cmd();
}
private void dl(string filename) {
using (var sftp = new SftpClient(this.Host, this.Port, this.Username, this.Password))
{
sftp.Connect();
using (var file = File.OpenWrite(this.LocalDestinationDIR + filename))
{
sftp.DownloadFile(this.RemoteFileDIR + filename, file);
}
sftp.Disconnect();
}
}
private void ssh_cmd() {
SshClient ssh = new SshClient(this.Host, this.Username, this.Password);
ssh.Connect();
string command = "cd /root && ls";
string[] str_result;
string str_results;
char ptn ='\n';
int i =0;
var result = ssh.RunCommand(command);
//MessageBox.Show(result.Result);
str_results = result.Result;
str_result = str_results.Split(ptn);
ssh.Disconnect();
for ( i = 0; i < str_result.Length; i++) {
//MessageBox.Show((str_result[i]));
if (str_result[i] != "")
{
dl(str_result[i]);
}
}
}
}
}
著者名
@taoka_toshiaki
※この記事は著者が30代前半に書いたものです.
Profile
高知県在住の@taoka_toshiakiです、記事を読んで頂きありがとうございます.
数十年前から息を吸うように日々記事を書いてます.たまに休んだりする日もありますがほぼ毎日投稿を心掛けています😅.
SNSも使っています、フォロー、いいね、シェア宜しくお願い致します🙇.
SNS::@taoka_toshiaki
タグ
Collections, ComponentModel, data, Drawing, Generic, Linq, Manager, net, NuGet, Package, SFTP, SSH, System, Tasks, Text, Threading, using, インストール, コード, ココ, こと, ご自分, ソース, ソリューション, ツール, パッケージ, マネージャー, ライブラリ, 下記, 使用, 入手, 拡張, 接続, 検索, 機能, 確立, 管理, 追加, 過程,