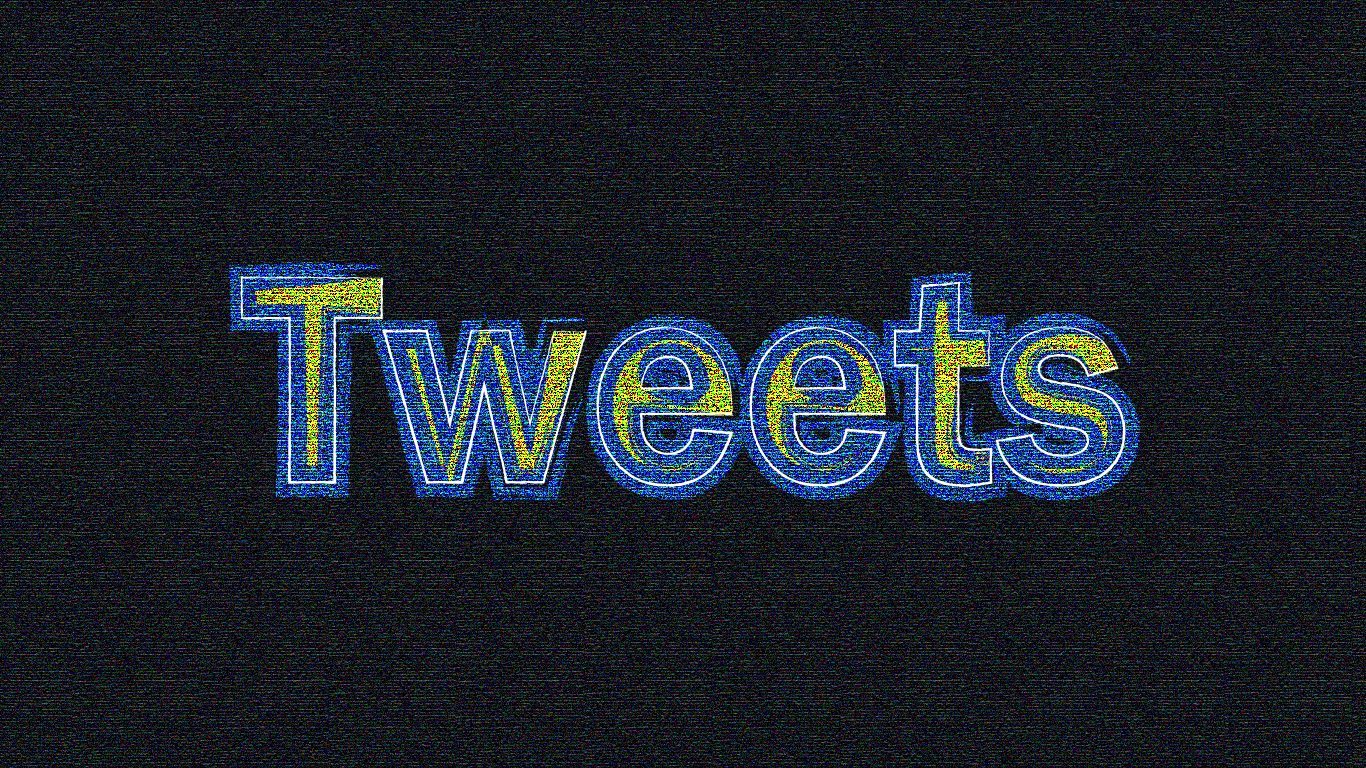
数珠繋ぎのツイートシステムに予約機能を付けました😂 #php #code
2022.10.07
おはようございます、偏頭痛持ちは雨が降るが一番大変です☔。
先日、数珠繋ぎのツイートシステムを作ったのですが、そのシステムに予約機能を付けました。尚、TwitterAPIのバージョン2でスケジュールのパラメーターが今のところ無いですね。これから先、機能が付くかも知れないですが今のところ無いようです。因みにソースコードは近日中にQiitaとGithubにUPします。此処ではソースコードの一部を掲載します(※記事を更新しました下へスクロール🫠)。
尚、crontabでPHPファイルを叩くようにしています、あと注意事項ですが予約を一度した投稿については変更等は出来ません、編集機能等の機能追加の予定はないです。また、予約管理はsqlite3を使用して管理しています。
<?php
date_default_timezone_set('Asia/Tokyo');
ini_set("display_errors",0);
require_once "./data/tw-config-v2.php";
require_once "../vendor/autoload.php";
use Abraham\TwitterOAuth\TwitterOAuth;
class tw
{
var $connection = null;
var $pdo = null;
function __construct()
{
$this->connection = new TwitterOAuth(APIKEY, APISECRET, ACCESSTOKEN, ACCESSTOKENSECRET);
$this->connection->setApiVersion("2");
}
function db_connection()
{
try {
//code...
$res = $this->pdo = new PDO("sqlite:./data/tw-tweets-db.sqlite3");
} catch (\Throwable $th) {
//throw $th;
//print $th->getMessage();
$res = false;
}
return $res;
}
function timecheck($timeonoff, $times)
{
if (!$timeonoff) return true;
$n = new DateTime();
$t = new DateTime($times);
return $t <= $n ? true : false;
}
function pickup_tweets(mixed $tw_text = null, int $timeonoff = 0, mixed $times = null, string $id = "")
{
if (!$times) return false;
$obj = (object)[];
$times = preg_replace("/\-/", "/", $times);
$times = preg_replace("/T/", " ", $times);
if ($this->timecheck($timeonoff, $times)) {
if (isset($tw_text) && is_array($tw_text)) {
foreach ($tw_text as $key => $value) {
if (preg_replace("/[ | ]/", "", $value)) {
$obj = !$key ? ($this->connection->post("tweets", ["text" => $value], true)
) : ($this->connection->post("tweets", ["reply" => ["in_reply_to_tweet_id" => $obj->data->id], "text" => $value], true)
);
}
}
return true;
}
} else {
return $timeonoff ? $this->save_sqlite($tw_text, $timeonoff, $times, $id): true;
}
}
function save_sqlite($tw_text = null, int $timeonoff = 0, mixed $times = null, string $id = "")
{
if ($this->db_connection()) {
try {
//code...
if (isset($tw_text) && is_array($tw_text)) {
foreach ($tw_text as $key => &$value) {
if (preg_replace("/[ | ]/", "", $value)) {
$stmt = $this->pdo->prepare("insert into tweets (tw_id,user,times,tw_text)values(:tw_id,:user,:times,:tw_text)");
$stmt->bindValue(":tw_id", $key, PDO::PARAM_INT);
$stmt->bindValue(":user", $id, PDO::PARAM_STR);
$stmt->bindValue(":times", $times, PDO::PARAM_STR);
$stmt->bindValue(":tw_text", $value, PDO::PARAM_STR);
$stmt->execute();
}
}
}
$this->pdo = null;
return true;
} catch (\Throwable $th) {
//throw $th;
return false;
}
}
}
function tweets_load(string $id = "")
{
if (!$id) return false;
try {
//code...
$value = null;
if ($this->db_connection()) {
$stmt = $this->pdo->prepare("select * from tweets where user = :user order by times,tw_id asc;");
$stmt->bindValue(":user", $id, PDO::PARAM_STR);
$res = $stmt->execute();
$value = $res ? $stmt->fetchAll() : false;
$this->pdo = null;
}
return $value;
} catch (\Throwable $th) {
//throw $th;
return false;
}
}
function tweets_update(int $key = 0, int $timeonoff = 0, mixed $times = null, string $id = "",mixed $tw_text="")
{
try {
//code...
if(!preg_replace("/[ | ]{0,}/","",$tw_text))return false;
if ($this->db_connection()) {
$stmt = $this->pdo->prepare("update tweets set tw_text = :tw_text where tw_id = :tw_id and user = :user and times = :times");
$stmt->bindValue(":tw_id", $key, PDO::PARAM_INT);
$stmt->bindValue(":user", $id, PDO::PARAM_STR);
$stmt->bindValue(":times", $times, PDO::PARAM_STR);
$stmt->bindValue(":tw_text", $tw_text, PDO::PARAM_STR);
$stmt->execute();
$this->pdo = null;
}
} catch (\Throwable $th) {
//throw $th;
return false;
}
return true;
}
function tweets_delete(int $key = 0, int $timeonoff = 0, mixed $times = null, string $id = "")
{
try {
//code...
if ($this->db_connection()) {
$stmt = $this->pdo->prepare("delete from tweets where tw_id = :tw_id and user = :user and times = :times");
$stmt->bindValue(":tw_id", $key, PDO::PARAM_INT);
$stmt->bindValue(":user", $id, PDO::PARAM_STR);
$stmt->bindValue(":times", $times, PDO::PARAM_STR);
$stmt->execute();
$this->pdo = null;
}
} catch (\Throwable $th) {
//throw $th;
return false;
}
return true;
}
function bat_tweets(mixed $value = null)
{
if (!$value) return false;
$obj = (object)[];
$t = "";
foreach ($value as $key => $val) {
if ($this->timecheck(1, $val["times"])) {
$obj = ($val["times"]<>$t)? ($this->connection->post("tweets", ["text" => $val["tw_text"]], true)
) : ($this->connection->post("tweets", ["reply" => ["in_reply_to_tweet_id" => $obj->data->id], "text" => $val["tw_text"]], true)
);
$this->tweets_delete($val["tw_id"], 1, $val["times"], $val["user"]);
$t = $val["times"];
} else {
// var_dump($val);
// break;
}
}
}
}
if ($argv[0]) {
$tw = new tw();
$value = $tw->tweets_load(xss_d($argv[1]));
$tw->bat_tweets($value);
}
function xss_d($val = false)
{
if (is_array($val)) {
foreach ($val as $key => $value) {
$val[$key] = strip_tags($value);
$val[$key] = htmlspecialchars($val[$key]);
}
} else {
$val = strip_tags($val);
$val = htmlspecialchars($val);
}
return $val;
}
追記:予約編集機能なども付けました🙄。
GithubとQiitaのリンクはこちらです。
Github:https://github.com/taoka-toshiaki/tweets-system-box1
Qiita:https://qiita.com/taoka-toshiaki/items/5ef12b60b267742bf584
著者名
@taoka_toshiaki
Profile
高知県在住の@taoka_toshiakiです、記事を読んで頂きありがとうございます.
数十年前から息を吸うように日々記事を書いてます.たまに休んだりする日もありますがほぼ毎日投稿を心掛けています😅.
SNSも使っています、フォロー、いいね、シェア宜しくお願い致します🙇.
SNS::@taoka_toshiaki
タグ
2, 3, 39, Asia, Code, crontab, date, default, github, ini, lt, php, qiita, Se, set, Sqlite, timezone, Tokyo, TwitterAPI, UP, コード, これ, システム, スクロール, スケジュール, ソース, ツイート, ところ, バージョン, パラメーター, ファイル, 一部, 下, 予定, 予約, 事項, 今, 使用, 偏頭痛, 先, 先日, 変更等, 大変, 投稿, 掲載, 数珠繋ぎ, 更新, 機能, 機能等, 此処, 注意, 管理, 編集, 記事, 近日, 追加, 雨,
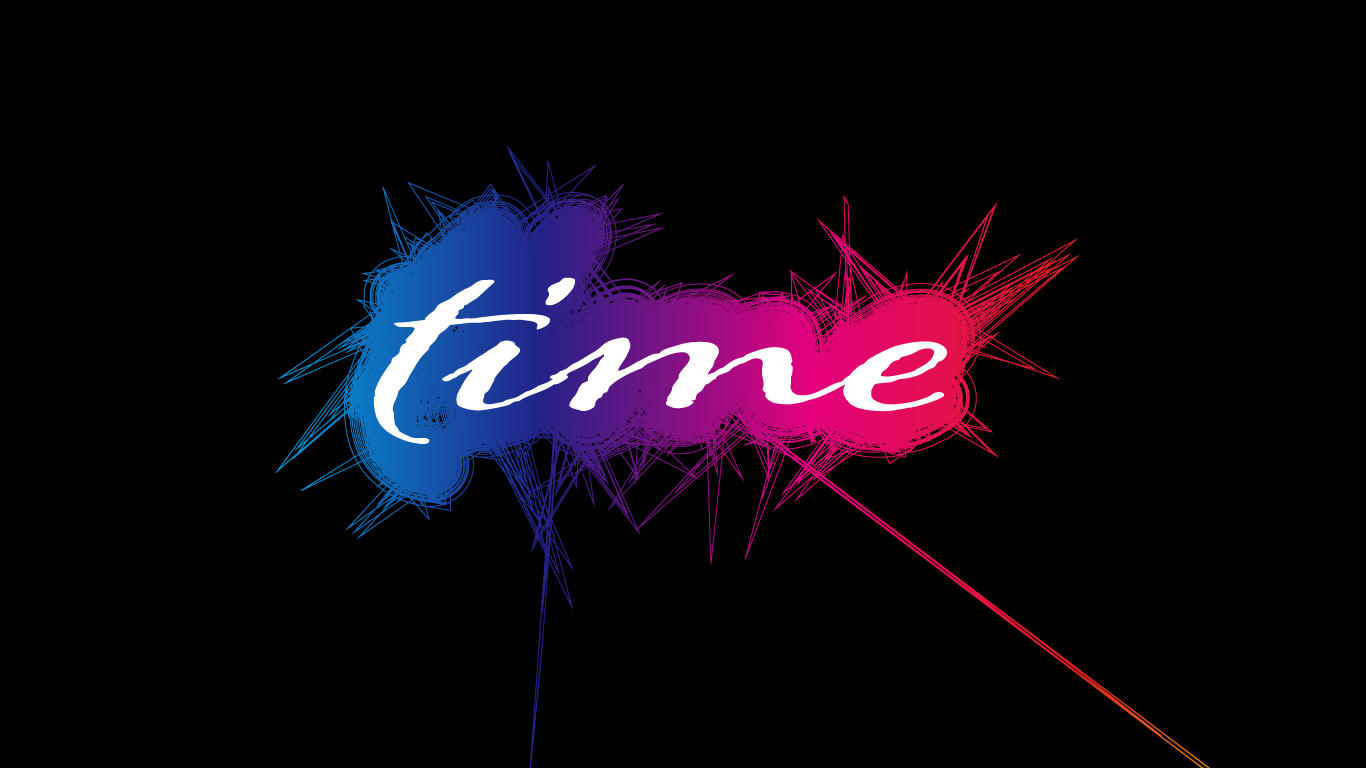
円安だから。貯金を進めてくるのは悪徳商法?
2022.07.12
おはようございます。NHKのYOUTUBE動画に「一見、悪徳に見えて、ただ貯金を勧めているだけの男たち」という動画あるのだけどアレ円安になってから見ると悪徳業者に見えてしょうがない・・・。
だからといって海外へ投資を進める記事ではないけれどね。地頭力がある人は10万円を元手にそれを何倍に増やすことが出来るそうです。株取引などはやってみると分かると思うけど、低いところで買って高くなりかけで売れば、その差額が利益になります。
但し、注意事項があってそういう事は誰でも出来るわけでもないみたいです。だから自分は投資信託をオススメします。特に人工知能(機械学習)が組み込まれている投資信託を使用すればそれなりに利益を得ることが出来ます。ここで重要なのはドルコスト平均法で買う(積立投資)という事が大事になります。あと追加資金を投入する時期なども大事です。
例えば何かの経済指標の発表がある前に買うのかそれとも発表後に買うのかなど、これは自分が下がりそうだな、上がりそうだなで買うタイミングを変えた方が良いです。投資信託でも同じで追加投資の資金は毎月同じ日にするよりか、自分で投資タイミングを考えて投資したほうが良いです。その方が利益に繋がりやすいです(同じことを2回書いているけど、其れぐらいタイミングは大事です)。
尚、前から言っているように投資・投機は自己責任ですので、、、以上、現場からでした。
著者名
@taoka_toshiaki
Profile
高知県在住の@taoka_toshiakiです、記事を読んで頂きありがとうございます.
数十年前から息を吸うように日々記事を書いてます.たまに休んだりする日もありますがほぼ毎日投稿を心掛けています😅.
SNSも使っています、フォロー、いいね、シェア宜しくお願い致します🙇.
SNS::@taoka_toshiaki
タグ
10, NHK, youtube, アレ, おすすめ, かけ, ここ, コスト, こと, これ, それ, ところ, ドル, わけ, 一見, 万, 事, 事項, 人, 人工, 但し, 何か, 何倍, 使用, 元手, 円安, 利益, 前, 動画, 商法, 地頭, 大事, 学習, 差額, 平均法, 悪徳, 投入, 投資, 投資信託, 指標, 時期, 株取引, 業者, 機械, 注意, 海外, 男, 発表, 知能, 積立, 経済, 自分, 記事, 誰, 貯金, 資金, 追加, 重要,
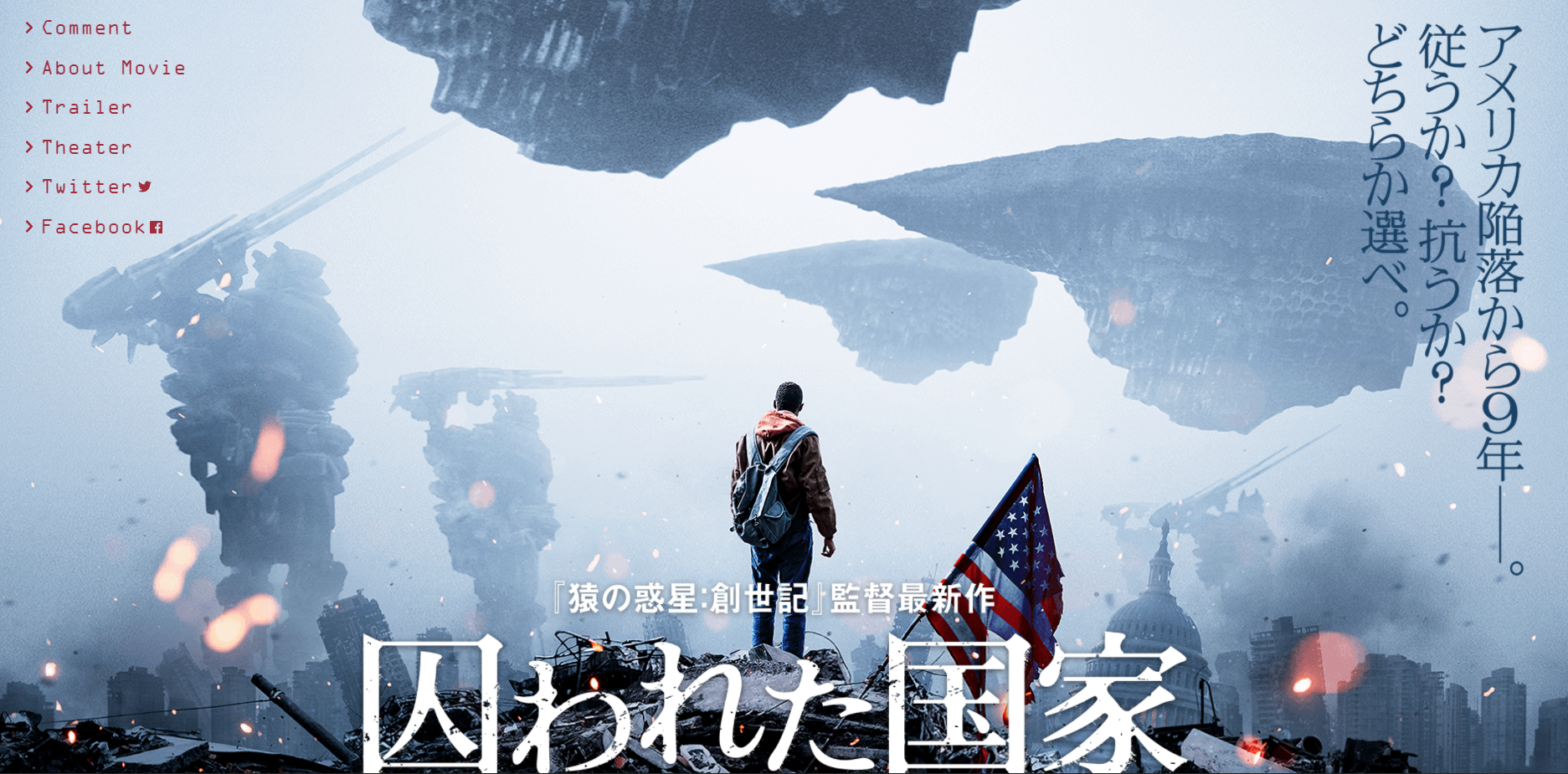
映画、囚われた国家を観ました。
2021.12.02
今日は昨日より寒いですね?
さて、囚われた国家という映画を遅れながら観ましたので、足跡的な感想を残しておきます。この映画、最後の最後まで見応えがありよかったです。まさにこの言葉がぴったりな映画「切り札は先に見せるな、見せるならさらに奥の手を持て」。そして、万人受けするかどうか分からない映画です・・・。
注意事項を言えば明るい映画ではないどちらかと言えばダークで知的な映画だと思ってください。これ以上言えばネタバレになると思いますのでラストは言えないけど・・・そうですね、全然ストーリーは違いますが映画、CUBEに近い何かがこの映画にはあるような気がします。
著者名
@taoka_toshiaki
Profile
高知県在住の@taoka_toshiakiです、記事を読んで頂きありがとうございます.
数十年前から息を吸うように日々記事を書いてます.たまに休んだりする日もありますがほぼ毎日投稿を心掛けています😅.
SNSも使っています、フォロー、いいね、シェア宜しくお願い致します🙇.
SNS::@taoka_toshiaki
タグ
CUBE, これ, ストーリー, ダーク, どちらか, ぴったり, ラスト, 万, 事項, 人受け, 今日, 何か, 切り札, 国家, 奥の手, 感想, 映画, 昨日, 最後, 気, 注意, 知的, 見応え, 言葉, 足跡,
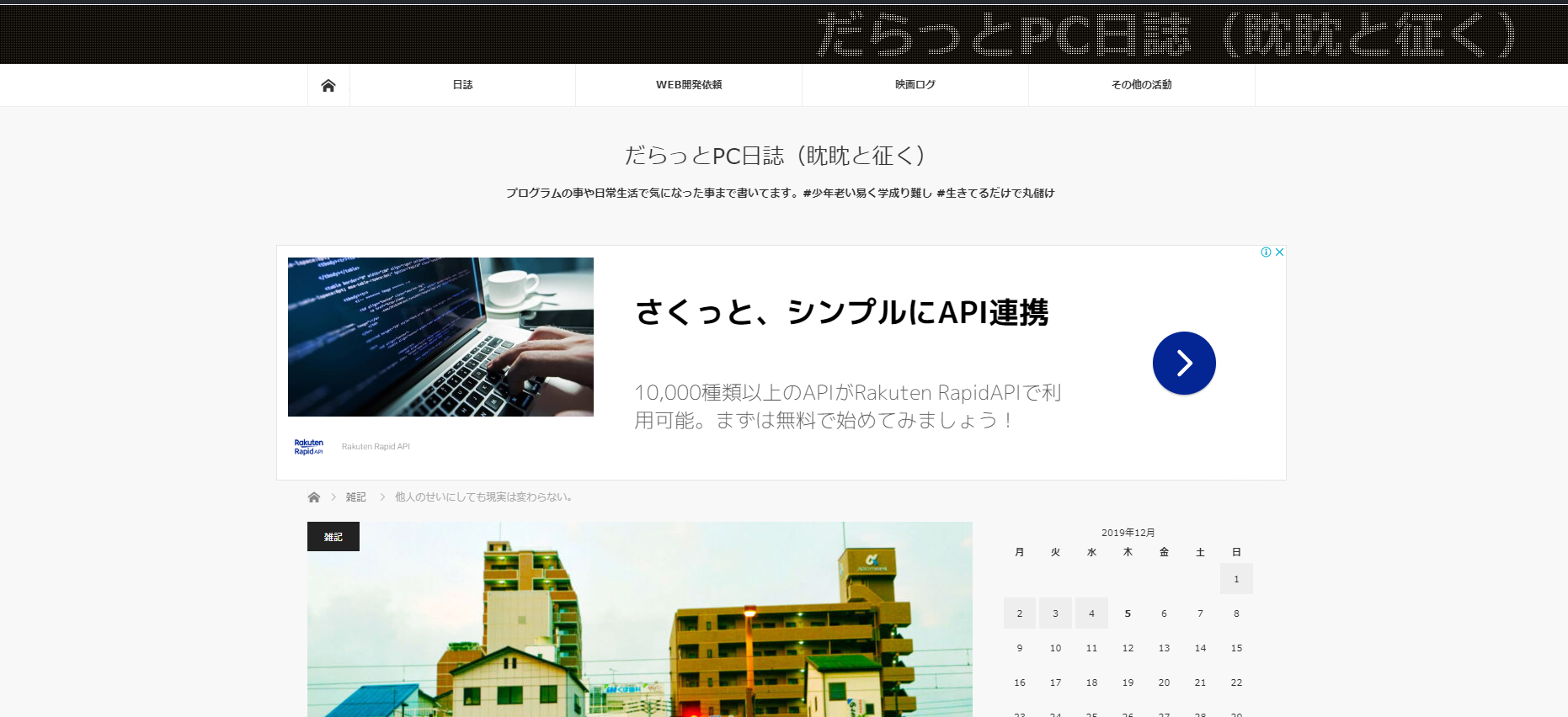
ワードプレス電光掲示板プラグインを作りました。
2019.12.05
電光掲示板(お知らせ)のプラグイン取扱に関して記載します。
プラグインに関してはワードプレス5.2以降を推奨としテーマファイル(外観)のheader.phpのbody直下に下記の記述が存在しない場合は追加記述を
お願いいたします。
<?php wp_body_open();?>
尚、プラグインのソースコードはご自由に変更頂き二次配布も構いませんが
ひとつ注意事項があります。参照した記事にリンクを貼ってください、
なお、事前の連絡等入りません。
電光掲示板(お知らせ)のソースコードは下記になります。
現在、テキストはHTMLタグを許していますので、いろいろと自由に
変更することが可能かと思います。
ちなみにこういった電光掲示板みたいな流れる仕様のコードは
インターネットの初期のころは多く見られましたが
このごろは全然、見なくなりましたね。。。
CSSの記述に関してはとくめいさんの記述を使用させて頂きました。
https://creatorclip.info/2014/06/css3-electric-bulletin-board/
とくめいさんも同じようなことを記事に記載しておりますが
自分もそのように感じました。
とくめいさんへ断りもなく使用してすみません、
Twitterで連絡しようかなと思ったのですが、それもなんだかと思い
勝手ながらこのような手段を取りました。
お知らせ(電光掲示板)のダウンロードは下記になります。
下記のファイルを解凍しワードプレスのプラグイン領域にフォルダごと
アップロードしプラグインを有効にするとご使用頂けます。
https://zip358.com/plugin/Z-Electric-bulletin-board.zip
<?php
/*
Plugin Name: Z-Electric-bulletin-board
Plugin URI: https://zip358.com/plugin/Z-Electric-bulletin-board.zip
Description: お知らせ
Author: taoka toshiaki
Version: 1.0
Author URI: https://zip358.com/
*/
add_action( 'wp_head', function() {
$color = get_option('ZEBB_color')?get_option('ZEBB_color'):"ffffff";
$cssdata ="
<style>
/* =====================
電光掲示板
======================= */
.ledText {
overflow: hidden;
position: relative;
padding:5px 0;
color: #$color;
font-size: 60px;
font-weight: bold;
background: #333333;
}
/* CSS3グラデーションでドット感を出す */
.ledText:after {
content: ' ';
display: block;
position: absolute;
top: 0;
right: 0;
bottom: 0;
left: 0;
background-image: linear-gradient(#0a0600 1px, transparent 0px), linear-gradient(0, #0a0600 1px, transparent 1px);
background-image: -webkit-linear-gradient(#0a0600 1px, transparent 0px), -webkit-linear-gradient(0, #0a0600 1px, transparent 1px);
background-size: 2px 2px;
z-index: 10;
}
/* CSS3アニメーションでスクロール */
.ledText span {
display: inline-block;
white-space: nowrap;
padding-left: 100%;
-webkit-animation-name: marquee;
-webkit-animation-timing-function: linear;
-webkit-animation-iteration-count: infinite;
-webkit-animation-duration: 15s;
-moz-animation-name: marquee;
-moz-animation-timing-function: linear;
-moz-animation-iteration-count: infinite;
-moz-animation-duration: 15s;
animation-name: marquee;
animation-timing-function: linear;
animation-iteration-count: infinite;
animation-duration: 15s;
}
@-webkit-keyframes marquee {
from { -webkit-transform: translate(0%);}
99%,to { -webkit-transform: translate(-100%);}
}
@-moz-keyframes marquee {
from { -moz-transform: translate(0%);}
99%,to { -moz-transform: translate(-100%);}
}
@keyframes marquee {
from { transform: translate(0%);}
99%,to { transform: translate(-100%);}
}
</style>
";
print $cssdata;
});
add_action("wp_body_open",function(){
$text = get_option('ZEBB_text')?get_option('ZEBB_text'):"";
if($text)print '<p class="ledText"><span>'.$text.'</span></p>';
});
add_action('admin_menu','Z_Electric_bulletin_board_set');
function Z_Electric_bulletin_board_set(){
add_options_page(
'zip358.com:プラグイン',
'電光掲示板設定',
'administrator',
'Z_Electric_bulletin_board',
function(){
if(isset($_POST["ZEBB_color"]) or isset($_POST["ZEBB_text"])){
$color = preg_match("/[a-zA-Z0-9]*/",$_POST["ZEBB_color"])?$_POST["ZEBB_color"]:"ffffff";
update_option('ZEBB_color', wp_unslash($color));
$text = $_POST["ZEBB_text"];
update_option('ZEBB_text', wp_unslash($text));
}
?>
<form method="post" action="">
<h2>電光掲示板設定</h2>
color code #<input type="text" style="width:350px" name="ZEBB_color" value="<?=get_option('ZEBB_color')?get_option('ZEBB_color'):""?>" placeholder="f7f7f7"><br>
text <input type="text" style="width:350px" name="ZEBB_text" value="<?=get_option('ZEBB_text')?get_option('ZEBB_text'):""?>" placeholder="文字を記入してください"><br>
テキスト文字を未入力にすると電光掲示板が表示されません
<?php submit_button(); ?>
</form>
<?php
}
);
}
著者名
@taoka_toshiaki
Profile
高知県在住の@taoka_toshiakiです、記事を読んで頂きありがとうございます.
数十年前から息を吸うように日々記事を書いてます.たまに休んだりする日もありますがほぼ毎日投稿を心掛けています😅.
SNSも使っています、フォロー、いいね、シェア宜しくお願い致します🙇.
SNS::@taoka_toshiaki
タグ
5.2, body, css, gt, header, html, lt, open, php, wp, いろいろ, インターネット, お知らせ, お願い, コード, こと, ごろ, ころ, ソース, タグ, テーマ, テキスト, トク, ひとつ, ファイル, プラグイン, プレス, メイ, リンク, ワード, 下記, 事前, 事項, 二, 仕様, 使用, 初期, 参照, 取扱, 可能, 場合, 変更, 外観, 存在, 推奨, 掲示, 注意, 現在, 直下, 自由, 記事, 記載, 記述, 追加, 連絡, 配布, 電光,