@Blog
日常日誌からプログラムやYOUTUBER紹介、旅日記まで日々更新中です。
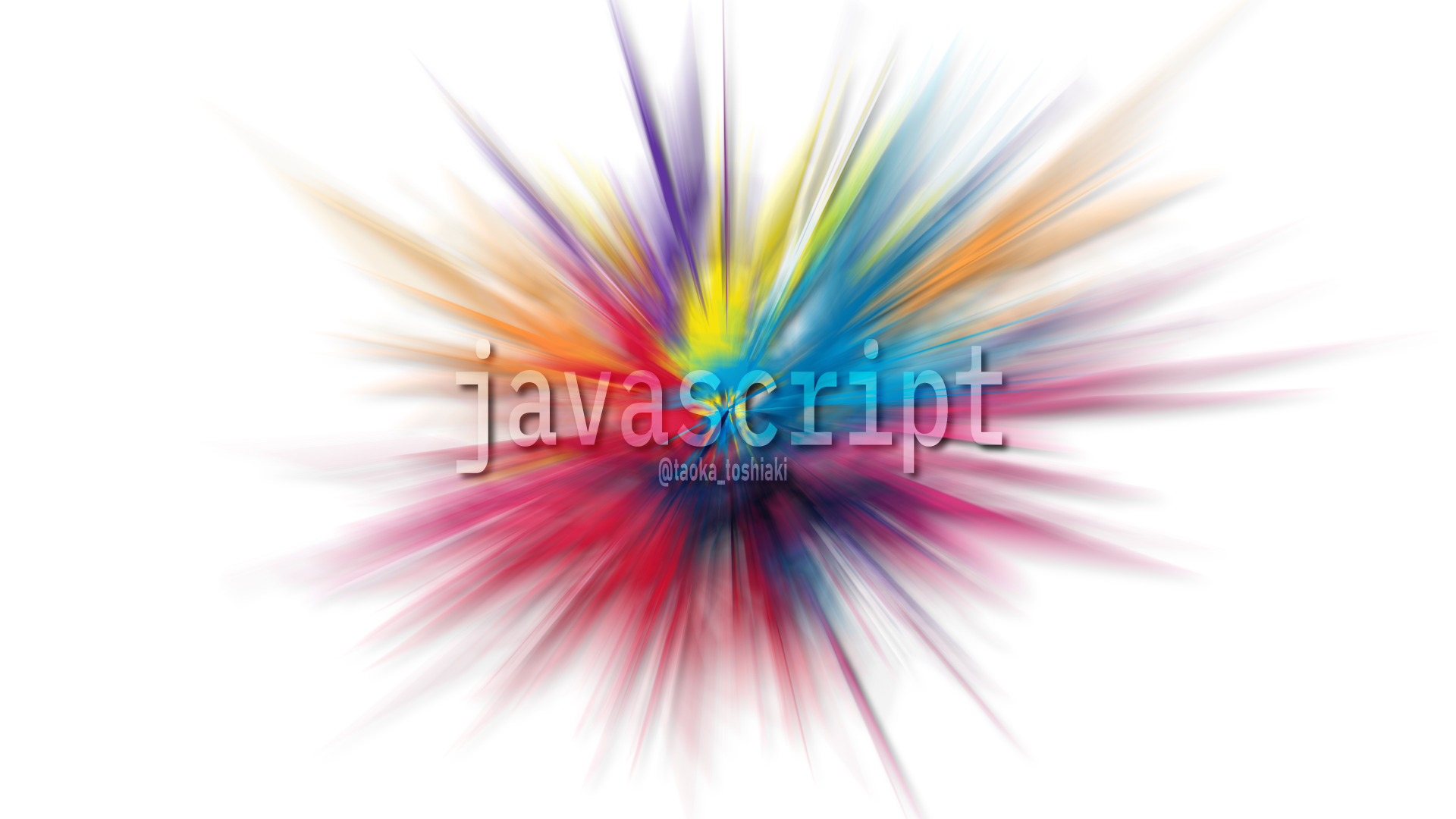
【#javascript定番】日付のカウントダウンを行う
2024.07.14
おはようございます.日付のカウントダウンを行うJavaScript言語の定番プログラムコードを作りましたので公開します.昨今、生成AIを使用してこのぐらいのコードは生成出来るかと思っていたのですが、こんなコードでも手直しが必要になりました.
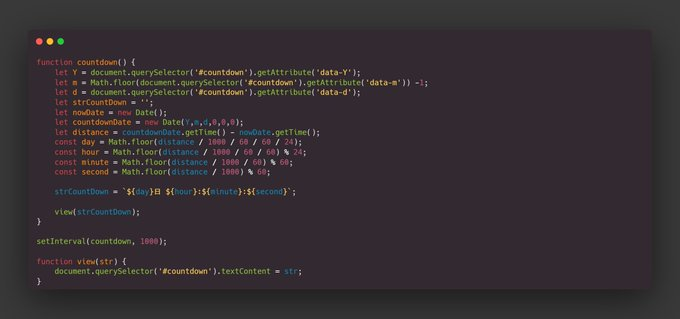
やはり今の生成AIは事細かい指示を出さないと上手く動かないような気がします.それはプログラミングじゃない事でもそんな感じなのかもしれません.
<h1 id="countdown" data-y="2025" data-m="07" data-d="11"></h1>
function countdown() {
let Y = document.querySelector('#countdown').getAttribute('data-Y');
let m = Math.floor(document.querySelector('#countdown').getAttribute('data-m')) -1;
let d = document.querySelector('#countdown').getAttribute('data-d');
let strCountDown = '';
let nowDate = new Date();
let countdownDate = new Date(Y,m,d,0,0,0);
let distance = countdownDate.getTime() - nowDate.getTime();
const day = Math.floor(distance / 1000 / 60 / 60 / 24);
const hour = Math.floor(distance / 1000 / 60 / 60) % 24;
const minute = Math.floor(distance / 1000 / 60) % 60;
const second = Math.floor(distance / 1000) % 60;
strCountDown = `${day}日 ${hour}:${minute}:${second}`;
view(strCountDown);
}
setInterval(countdown, 1000);
function view(str) {
document.querySelector('#countdown').textContent = str;
}
デモページはこちらになります.
https://zip358.com/tool/demo94
明日へ続く.
著者名
@taoka_toshiaki
※この記事は著者が40代前半に書いたものです.
Profile
高知県在住の@taoka_toshiakiです、記事を読んで頂きありがとうございます.
数十年前から息を吸うように日々記事を書いてます.たまに休んだりする日もありますがほぼ毎日投稿を心掛けています😅.
SNSも使っています、フォロー、いいね、シェア宜しくお願い致します🙇.
SNS::@taoka_toshiaki
タグ
countdown, countdownDate.getTime, data-y, distance, document.querySelector, floor, getAttribute, gt, hour, let, lt, minute, nowDate.getTime, querySelector, quot, second, setInterval, STR, strCountDown, textContent,
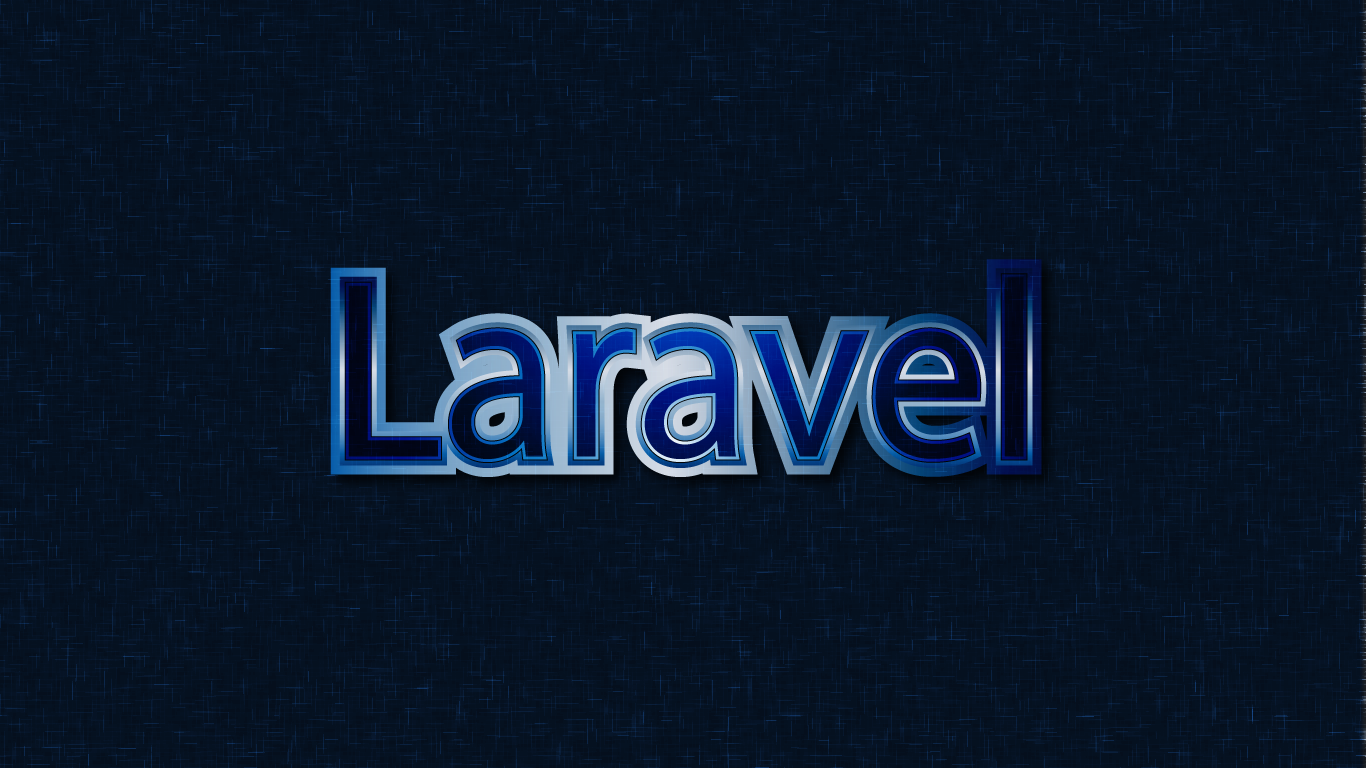
laravelのFactoryって便利なダミーデータが作れるよ。
2023.09.20
おはようございます、laravelのFactoryって便利なダミーデータが作れるよ。ユニットテストするときに使用するのでLaravelを触ったことがある人なら分かると思いますが、ダミーデータ作るれるのは便利ですよねぇーーー😌。因みに公式サイトのサンプルコードに細工したサンプルコードを載せておきます。
namespace Database\Factories;
use Illuminate\Database\Eloquent\Factories\Factory;
use Illuminate\Support\Str;
use App\zip358com;
class UserFactory extends Factory
{
public function definition()
{
return [
'name' => $this->faker->name(),
'email' => $this->faker->unique()->safeEmail(),
'email_verified_at' => now(),
'password' => '$2y$10$92IXUNpkjO0rOQ5byMi.Ye4oKoEa3Ro9llC/.og/at2.uheWG/igi', // password
'remember_token' => Str::random(10),
'zip358com' =>function(){
return (zip358com::first())->url;
}
];
}
}
最後に記載していることが恐らくテストを行っていくうちに必要になってくる事だと思います。もう一つ必要になってくるのは、固定したデータでユニットテストを行いたい場合はユニットテストの方でこのような感じに書くと良いです。
use Illuminate\Foundation\Testing\DatabaseMigrations;
use Tests\TestCase;
use App\User;
class YourTest extends TestCase
{
use DatabaseMigrations;
public function test_example()
{
$user = User::factory()->create([
'name' =>'zip 358',
'email' => 'mail@zip358.com',
'email_verified_at' => now(),
'password' => '1234567890', // password
'remember_token' => Str::random(10),
'zip358com'=>'https://zip358.com',
]);
$this->assertEquals('zip 358', $user->name);
$user->delete();
}
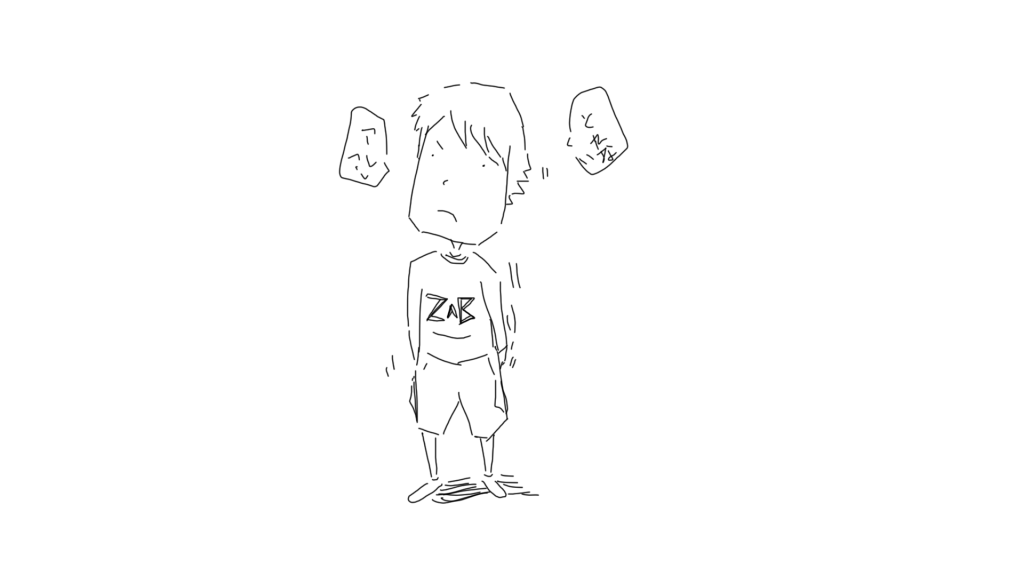
著者名
@taoka_toshiaki
※この記事は著者が40代前半に書いたものです.
Profile
高知県在住の@taoka_toshiakiです、記事を読んで頂きありがとうございます.
数十年前から息を吸うように日々記事を書いてます.たまに休んだりする日もありますがほぼ毎日投稿を心掛けています😅.
SNSも使っています、フォロー、いいね、シェア宜しくお願い致します🙇.
SNS::@taoka_toshiaki
タグ
Factory, FIRST, function, gt, Laravel, namespace DatabaseFactories, Now, Og-, password, random, remember_token, return, STR, use IlluminateDatabaseEloquentFactoriesFactory, use IlluminateFoundationTestingDatabaseMigrations, use IlluminateSupportStr, use TestsTestCase, user, ユニットテスト,
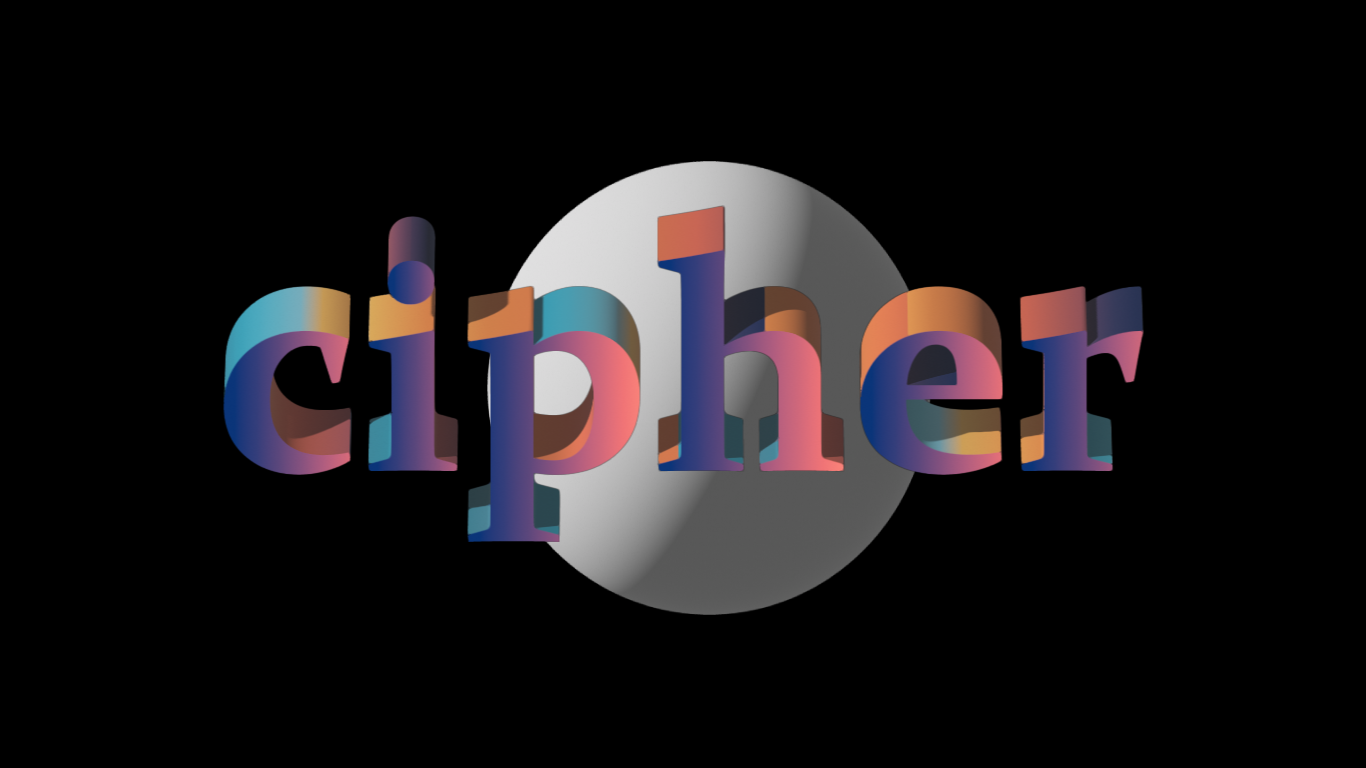
ブルータス、お前もか?古代の暗号シーザー。 #phpcode
2023.04.30
おはようございます。古代にも暗号というものがあったらしい。古代の人が使っていたシーザーという暗号をPHP化しました。demo74のページを見ると実行結果が表示されていると思います。
古代にはPCというものが無かったので、これでも解読するのにある程度、時間がかかったんでしょうね。ぱっと見、暗号化されているのは分かるけど瞬時に解読できる人はあまりいなかっただと思います。近年では量子暗号とか、パッと見どころかPCがあっても鍵が無いと解読に途方も無い時間を費やする暗号までありますよね。そう思うと暗号の歴史を辿るのも面白いかもしれないですね。
<?php
function caesarCipher($str, $shift) {
$result = "";
$len = strlen($str);
// 26文字のアルファベットを配列として定義する
$alpha = range('a', 'z');
for ($i = 0; $i < $len; $i++) {
$char = strtolower($str[$i]); // 大文字を小文字に変換する
if (in_array($char, $alpha)) { // アルファベットの場合のみシフトする
$index = array_search($char, $alpha); // アルファベットの位置を検索する
$newIndex = ($index + $shift) % 26; // シフト後のアルファベットの位置を計算する
$result .= $alpha[$newIndex]; // シフト後のアルファベットを結果に追加する
} else {
$result .= $char; // アルファベット以外はそのまま結果に追加する
}
}
return $result;
}
// 使用例
$plaintext = "hello world";
$ciphertext = caesarCipher($plaintext, 3);
echo $ciphertext; // "khoor zruog"
著者名
@taoka_toshiaki
※この記事は著者が40代前半に書いたものです.
Profile
高知県在住の@taoka_toshiakiです、記事を読んで頂きありがとうございます.
数十年前から息を吸うように日々記事を書いてます.たまに休んだりする日もありますがほぼ毎日投稿を心掛けています😅.
SNSも使っています、フォロー、いいね、シェア宜しくお願い致します🙇.
SNS::@taoka_toshiaki
タグ
$alpha, $ciphertext, $len, $newIndex, $plaintext, $shift, caesarCipher, char, echo, else, lt, quot, range, result, return, STR, strlen, シーザー, 小文字, 解読,
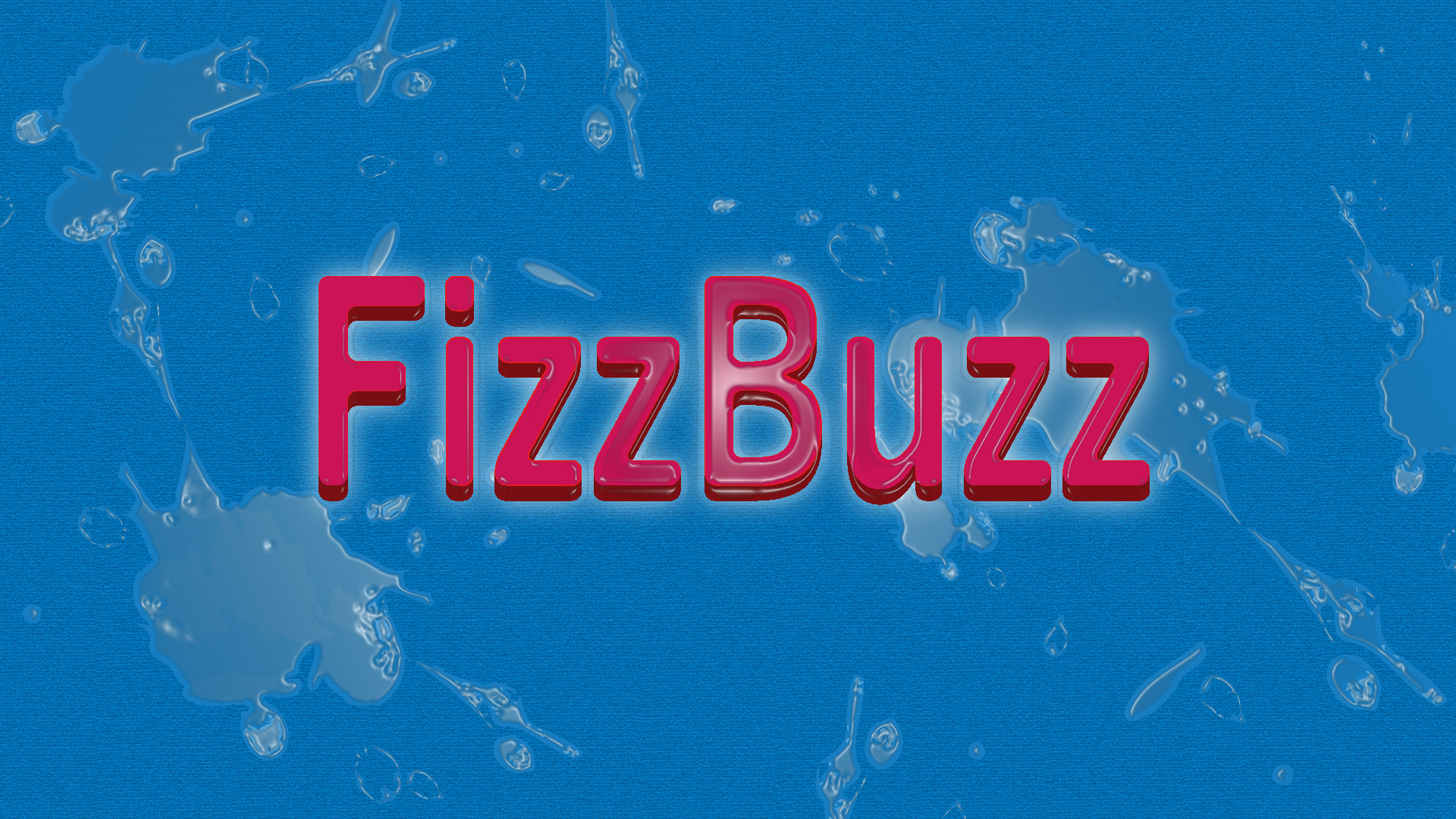
現役エンジニアならFizzBuzz問題なんて余裕なのか検証してみたをやってみた?
2021.03.22
【現役エンジニアならFizzBuzz問題なんて余裕なのか検証してみたをやってみた】、この道、10年選手の自分が解いてみた結果、2つの解になることになりました。でも自分はかなり緊張するタイプなのでこういう場ではなんかミスりそうです。2つの解になる理由は15の時、5の倍数でも3の倍数でもあり15の倍数でもあるからです。これに疑問をもつはずなのですが・・・。皆、言わなかったところが日本人的だなと感じました。因みに自分はこういう疑問を言うタイプです、なので衝突が上と起こるのかなと思います・・・。
尚、自分は3分ぐらいです。コードを書くより誰かに書いてもらいたい(^_^;)、もっとタイピングが早くなれば2分ぐらいで書けると思います?ではおつおつでした。
<?php
$ary1 = [];
$check1 = function ($hoge) {
$str = [];
if ($hoge % 3 === 0) {
$str[] = "Fizz($hoge)";
}
if ($hoge % 5 === 0) {
$str[] = "Buzz($hoge)";
}
if ($hoge % 15 === 0) {
$str[] = "FizzBuzz($hoge)";
}
if (!count($str)) {
$str[] = $hoge;
}
return implode(",",$str);
};
$ary2 = [];
$check2 = function ($hoge) {
$str = [];
if ($hoge % 15 === 0) {
$str[] = "FizzBuzz($hoge)";
}elseif($hoge % 5 === 0){
$str[] = "Buzz($hoge)";
}elseif($hoge % 3 === 0){
$str[] = "Fizz($hoge)";
}
if (!count($str)) {
$str[] = $hoge;
}
return implode(",",$str);
};
for ($i = 1; $i <= 100; $i++) {
$ary1[($i-1)] = $check1($i);
$ary2[($i-1)] = $check2($i);
}
print implode(",", $ary1).PHP_EOL;
print implode(",", $ary2).PHP_EOL;
著者名
@taoka_toshiaki
※この記事は著者が40代前半に書いたものです.
Profile
高知県在住の@taoka_toshiakiです、記事を読んで頂きありがとうございます.
数十年前から息を吸うように日々記事を書いてます.たまに休んだりする日もありますがほぼ毎日投稿を心掛けています😅.
SNSも使っています、フォロー、いいね、シェア宜しくお願い致します🙇.
SNS::@taoka_toshiaki
タグ
1, 10, 15, 2, 3, 5, ary, check, FizzBuzz, function, hog, hoge, if, lt, php, STR, エンジニア, かなり, コード, こと, これ, ダイビング, タイプ, ところ, パス, ミス, りそう, 上, 余裕, 倍数, 問題, 場, 日本人, 時, 検証, 現役, 理由, 疑問, 皆, 結果, 緊張, 自分, 衝突, 解, 誰か, 道, 選手,
もし今、自分が小学生だったら自由研究でこんなの作ってると思いたい。
2019.08.24
もし今、自分が小学生だったら自由研究でこんなの作ってると思いたい。
自動で九九表を生成するjQueryなんかを作ってそう。
(いまの小学生が羨ましいなw夏休み一日中ネットに浸っているだろう)
デモサイトはこちら
https://zip358.com/tool/demo5/index-12.php
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>九九表</title>
<link rel="stylesheet" href="../MDB-Free_4.8.5/css/bootstrap.css" >
<link rel="stylesheet" href="../MDB-Free_4.8.5/css/mdb.css" >
<link rel="stylesheet" href="../fontawesome-free-5.9.0-web/css/all.css">
<script src="../jquery/jquery-3.4.1.js"></script>
</head>
<body>
<form>
<div class="form-group">
<label for="exampleInputEmail1">数字を入力してください</label>
<input type="text" class="form-control" id="inp" aria-describedby="inp" placeholder="数字を入力してください(デフォルト(9))">
<small id="emailHelp" class="form-text text-muted">1以下の数字、数字以外、または桁が2桁を超えた場合デフォルトを表示します</small>
</div>
</form>
<span id="tbl99"></span>
<script>
$(function(){
var cc = function(){
var c=$(this).val().match(/[0-9]{1,2}/) && Number($(this).val())>1?$(this).val():9;
$("#tbl99").html(c99(c));
return c;
};
$("#tbl99").html(function(){
c99($("#inp").val(cc));
});
$("#inp").on("keyup",cc);
$("#inp").on("keydown",cc);
});
function c99(c){
var str="<h1><span class="badge badge-primary">九九表</span></h1>";
var x = y = c;
str+="<table class="table table-striped table-dark table-bordered">";
for(var i=1;i<=x;i++){
str+="<tr>";
for(var ii=1;ii<=y;ii++){
var s = i * ii;
str+="<td>" + i + " × " + ii + " = " + s + "</td>";
}
str+="</tr>";
}
str+="</table>";
return str;
}
</script>
</body>
</html>
著者名
@taoka_toshiaki
※この記事は著者が30代前半に書いたものです.
Profile
高知県在住の@taoka_toshiakiです、記事を読んで頂きありがとうございます.
数十年前から息を吸うように日々記事を書いてます.たまに休んだりする日もありますがほぼ毎日投稿を心掛けています😅.
SNSも使っています、フォロー、いいね、シェア宜しくお願い致します🙇.
SNS::@taoka_toshiaki
タグ
aria-describedby, cc, content, css, device-width, function, IE, Ii, initial-scale, inp", jquery, lt, match, Number, placeholder, quot, STR, stylesheet", val,
変なコードを書いてしまった。それはいつものこと(笑)
2019.08.10
変なコードを書いてしまった。
PHPにstr_repeatという関数が存在しているのだけど
何のために使用するのか全然わからない。関数の内容は任意の文字を
指定回数、繰り返した文字列として返してくれるというものです。
試しにその関数を使用し変なコードを書きました。
茶目っ気ですので…。これがPCに負担がかかるとか
無限ループとかの処理にすると御縄なんでしょうけど・・・。
サンプルコードはこちらから
https://zip358.com/tool/demo5/index-11.php
<!DOCTYPE html>
<!--[if lt IE 7]> <html class="no-js lt-ie9 lt-ie8 lt-ie7"> <![endif]-->
<!--[if IE 7]> <html class="no-js lt-ie9 lt-ie8"> <![endif]-->
<!--[if IE 8]> <html class="no-js lt-ie9"> <![endif]-->
<!--[if gt IE 8]><!--> <html class="no-js"> <!--<![endif]-->
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title></title>
<meta name="description" content="">
<meta name="viewport" content="width=device-width, initial-scale=1">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<script src="../jquery.tubular.1.0.1/js/jquery.tubular.1.0.js"></script>
<link rel="stylesheet" type="text/css" href="../jquery.tubular.1.0.1/css/screen.css">
<script>
$('document').ready(function() {
var options = {
videoId: '760lRwLKFF0',
mute: true,
};
$('#bgmovie').tubular(options);
});
</script>
<style>
body{
background-color: #000;
color: #fff;
font-size: 80px;
line-height: 80px;
}
p{
color: #38a9c5;
}
</style>
</head>
<body>
<div id="bgmovie">
<!--[if lt IE 7]>
<p class="browsehappy">You are using an <strong>outdated</strong> browser. Please <a href="#">upgrade your browser</a> to improve your experience.</p>
<![endif]-->
<?php
$str = str_repeat("高知 よさこい 踊る,",7);
var_dump(str_getcsv($str));
?>
<p class="oshite" data-oshite="<?=$str?>"style="text-decoration: underline">▼おして知るべし</p>
※おして知るべしをクリックすると7ウィンドウ開きますよ!!
<?php
foreach(str_getcsv($str) as $val){
?>
<?php
}
?>
</div>
<script>
$(function(){
$(".oshite").on("click",function(){
let oshite = $(this).attr("data-oshite").split(",");
for(var i = 0 ; i < oshite.length ; i++){
if(oshite[i]!==""){
window.open("https://twitter.com/search?src=typed_query&q=" + encodeURIComponent(oshite[i]));
}
}
});
});
</script>
<script src='https://vjs.zencdn.net/7.6.0/video.js'></script>
</body>
</html>
著者名
@taoka_toshiaki
※この記事は著者が30代前半に書いたものです.
Profile
高知県在住の@taoka_toshiakiです、記事を読んで頂きありがとうございます.
数十年前から息を吸うように日々記事を書いてます.たまに休んだりする日もありますがほぼ毎日投稿を心掛けています😅.
SNSも使っています、フォロー、いいね、シェア宜しくお願い致します🙇.
SNS::@taoka_toshiaki
タグ
--, 11, 358, 5, 7, 8, 9, class, com, demo, DOCTYPE, endif, gt, html, IE, if, index, lt, lt-ie, no-js, PC, php, repeat, STR, tool, zip, いつも, コード, こちら, こと, これ, サンプル, それ, ため, もの, ループ, 任意, 何, 使用, 内容, 処理, 回数, 変, 存在, 指定, 文字, 文字列, 無限, 縄, 茶目っ気, 負担, 関数,
#プログラムサンプル ブログパーツは検索して出てくるのでうっぷします。
2015.03.11
ブログパーツの簡素な仕組みをUPします。基本はこれだけです。基本はこれだけですけど応用すると少々面倒くさいのでそこら辺はご想像におまかせします。これを基にして正規に配布する状態にするには、サーバの負荷の事やセキュリティとかそういう事まで考えなくてはならないので少々面倒くさいです。特にIDを発行して配布する場合などは結構、コードを書かないといけないです。また、悪意のあるユーザーがサーバに負荷をかけれる要因にもなりますので、安易にブログパーツを提供するのはオススメできません。ちなみに自分のブログパーツ用のJSを貼り付けても動作はしません(ファイルがないので)。ただ、下記のサンプルコードを自分自身のサーバ上に設置し、設置したURL(任意のURL)をJSで呼び出すと動作し、それを配布することも可能です。
PHPファイル
<?php header("Content-type: application/javascript"); $str = htmlspecialchars($_GET["hoge"]); if($str!=""){ echo "document.write($str);"; }else{ echo "document.write('hoge!?');"; } ?>
JS貼付け用。
<script src="https://zip358.com/tool/sample-tool/sample-blogtool.php?hoge='こんにちは'" language="JavaScript" charset="utf-8"></script>
著者名
@taoka_toshiaki
※この記事は著者が30代前半に書いたものです.
Profile
高知県在住の@taoka_toshiakiです、記事を読んで頂きありがとうございます.
数十年前から息を吸うように日々記事を書いてます.たまに休んだりする日もありますがほぼ毎日投稿を心掛けています😅.
SNSも使っています、フォロー、いいね、シェア宜しくお願い致します🙇.
SNS::@taoka_toshiaki
タグ
AM, charset, Content-type, document.write, echo, header, hoge, htmlspecialchars, javascript, JS貼付け用, script src, script>, STR, utf-8, これだけ, ご想像, サーバ, セキュリティ, そこら辺, プログラムサンプル ブログパーツ, 負荷,